Developer Guide
Table of Contents
- Table of Contents
- Acknowledgements
- Setting up, getting started
- Design
- Documentation, logging, testing, configuration, dev-ops
- Planned Enhancements
- Appendix: Requirements
- Appendix: Instructions for manual testing
- Appendix: Effort
Acknowledgements
- HRConnect is a brownfield project based on AddressBook Level-3 (UG, DG).
- Certain parts of
Project
andAssignment
related features contain altered code from the original AddressBook Level-3 (UG, DG). - Parts of the User Guide and Developer Guide of HRConnect are based on those for the original AddressBook Level-3 (UG, DG).
Setting up, getting started
Refer to the guide Setting up and getting started.
Design

.puml
files used to create diagrams in this document are located in the docs/diagrams
folder. Refer to the PlantUML Tutorial at se-edu/guides to learn how to create and edit diagrams.
Architecture
The Architecture Diagram given above explains the high-level design of the App.
Given below is a quick overview of main components and how they interact with each other.
Main components of the architecture
The component Main
(consisting of classes Main
and MainApp
) is in charge of the app launch and shut down.
- At app launch, it initializes the other components in the correct sequence, and connects them up with each other.
- At shut down, it shuts down the other components and invokes cleanup methods where necessary.
The bulk of the app’s work is done by the following four components:
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
Commons
represents a collection of classes used by multiple other components.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete 1
.
Each of the four main components (also shown in the diagram above),
- defines its API in an
interface
with the same name as the Component. - implements its functionality using a concrete
{Component Name}Manager
class which follows the corresponding APIinterface
mentioned in the previous point.
For example, the Logic
component defines its API in the Logic.java
interface and implements its functionality using the LogicManager.java
class which follows the Logic
interface. Other components interact with a given component through its interface rather than the concrete class (reason: to prevent outside component’s being coupled to the implementation of a component), as illustrated in the (partial) class diagram below.
The sections below give more details of each component.
UI component
The API of this component is specified in Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, EmployeeListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class which captures the commonalities between classes that represent parts of the visible GUI.
The UI
component uses the JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- executes user commands using the
Logic
component. - listens for changes to
Model
data so that the UI can be updated with the modified data. - keeps a reference to the
Logic
component, because theUI
relies on theLogic
to execute commands. - depends on some classes in the
Model
component, as it displaysEmployee
object residing in theModel
.
Logic component
API : Logic.java
Here’s a (partial) class diagram of the Logic
component:
The sequence diagram below illustrates the interactions within the Logic
component, taking execute("delete 1")
API call as an example.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline continues till the end of diagram.
How the Logic
component works:
- When
Logic
is called upon to execute a command, it is passed to anAddressBookParser
object which in turn creates a parser that matches the command (e.g.,DeleteCommandParser
) and uses it to parse the command. - This results in a
Command
object (more precisely, an object of one of its subclasses e.g.,DeleteCommand
) which is executed by theLogicManager
. - The command can communicate with the
Model
when it is executed (e.g. to delete an employee).
Note that although this is shown as a single step in the diagram above (for simplicity), in the code it can take several interactions (between the command object and theModel
) to achieve. - The result of the command execution is encapsulated as a
CommandResult
object which is returned back fromLogic
.
Here are the other classes in Logic
(omitted from the class diagram above) that are used for parsing a user command:
How the parsing works:
- When called upon to parse a user command, the
AddressBookParser
class creates anXYZCommandParser
(XYZ
is a placeholder for the specific command name e.g.,AddCommandParser
) which uses the other classes shown above to parse the user command and create aXYZCommand
object (e.g.,AddCommand
) which theAddressBookParser
returns back as aCommand
object. - All
XYZCommandParser
classes (e.g.,AddCommandParser
,DeleteCommandParser
, …) inherit from theParser
interface so that they can be treated similarly where possible e.g, during testing.
Model component
API : Model.java
The Model
component,
- stores the address book data i.e., all
Employee
objects (which are contained in aUniqueEmployeeList
object). - stores the currently ‘selected’
Employee
objects (e.g., results of a search query) as a separate filtered list which is exposed to outsiders as an unmodifiableObservableList<Employee>
that can be ‘observed’ e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. - stores a
UserPref
object that represents the user’s preferences. This is exposed to the outside as aReadOnlyUserPref
objects. - stores a
CommandTextHistory
object that represents the user’s command history. - does not depend on any of the other three components (as the
Model
represents data entities of the domain, they should make sense on their own without depending on other components)

Tag
list and a Skill
list in the AddressBook
, which Employee
references. This allows AddressBook
to only require one Tag
object per unique tag, instead of each Employee
needing their own Tag
objects. Likewise, AddressBook
only requires one Skill
object per unique skill, instead of each Employee
needing their own Skill
objects.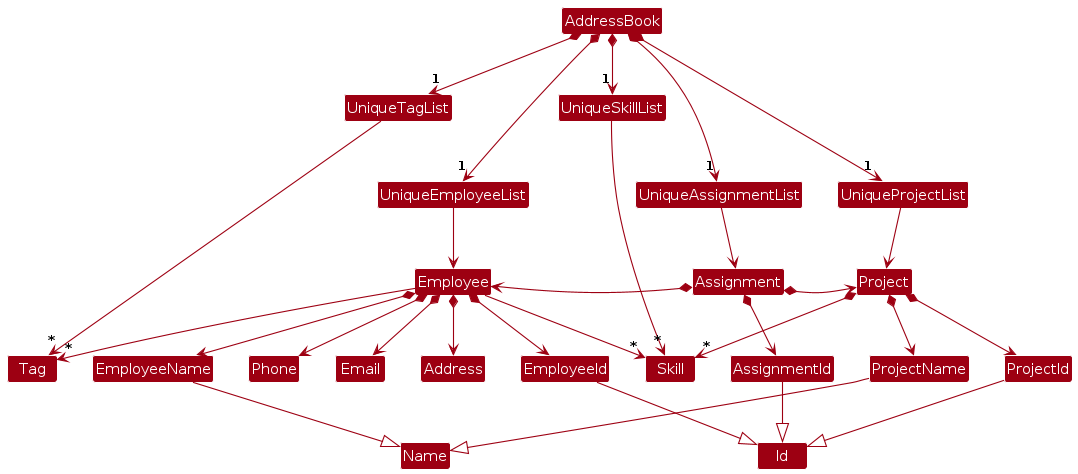
Storage component
API : Storage.java
The Storage
component,
- can save the address book data, user preference data and command text history in JSON format, and read them back into corresponding objects.
- inherits from both
AddressBookStorage
,UserPrefStorage
andCommandTextHistoryStorage
, which means it can be treated as either one (if only the functionality of only one is needed). - depends on some classes in the
Model
component (because theStorage
component’s job is to save/retrieve objects that belong to theModel
)
Common classes
Classes used by multiple components are in the seedu.address.commons
package.
Documentation, logging, testing, configuration, dev-ops
Planned Enhancements
Team size: 5
- Improve UI resizing: Currently, with smaller window sizes (including default size of window on first startup), part of the UI can be cut off. We plan to improve UI dynamic resizing to support more window sizes.
- Improve UI design: The current UI is functional but lacking in aesthetics. We plan to redesign the UI to improve readability and reduce confusion for users.
-
Clear all assignments feature: We plan to add a
clearassignments
command which allows users to delete all assignments. - Make sample data more relevant: Some of the example commands and sample data contain information that are not relevant to human resource management tasks. (For instance, some employees are tagged as ‘friends’, ‘family’, etc.) We plan to replace these with more fitting examples.
-
Allow users to specify other fields in
listprojectmembers
: Currently, users can only specify project name, but this could result in project members from multiple projects with the same name being shown. We plan to improve this command by allowing users to specify fields such as project ID instead, such aslistprojectmembers pid/1
. Since project IDs uniquely identify a project, users will be able to see only project members from that project specified. -
Improved email validation: Currently, users can enter emails without a top-level domain, such as
example@email
. We plan to validate emails to require that a top-level domain be specified. Thus, emails such asjohn@email.com
will be allowed, butjohn@email
will not be. -
Update UI after
assign
: Currently, when users executelistprojectmembers
and then executeassign
, the Assignments panel may still continue to show the filtered list of assignments. We plan to clear existing filters after eachassign
command, so users can see the full list of assignments. -
Allow users to specify both list index or ID for certain commands: For some commands such as
delete
, the list index (position of the item in display list) is used. However, for other commands such asassign
, ID is used. For flexibility and to reduce confusion, we plan to let users specify which to use through prefixes (pid/
,id/
,li/
(list index) etc.) -
Improve formatting of command success / error messages: Certain success messages (such as that for the
assign
command) are too long / go off-screen and become hard to read. We plan to format these messages better to increase readability. -
Better error message for employee / project / assignment IDs that are too large: As IDs are currently stored as
int
, storing IDs with too large of a number (e.g. ten digits of ‘9’) leads to overflow. However, the error message only reminds the user they should use numeric IDs. We plan to update the error message to mention the numerical limit to IDs.
Appendix: Requirements
Product scope
Target user profile:
- Project managers of a tech company with many employees with different skill sets and roles
- works in a team of other project managers
- manages recruitment and manpower allocation for company projects
- has a need to manage a significant number of contacts
- prefer desktop apps over other types
- can type fast
- prefers typing and keyboard shortcuts to mouse interactions
- is reasonably comfortable using and prefers CLI apps
Value proposition:
HRConnect provides fast access to employee, project, and candidate contact details, optimized for project managers who prefer a CLI. It allows talent pool organization, project management, and manpower allocation, all through a streamlined, command-based interface designed for speed and efficiency.
User stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
* * * |
new user | see usage instructions and tips | refer to instructions and tips when I forget how to use the App |
* * * |
manager / lead | add a new employee | |
* * * |
project manager | add a new project | |
* * * |
manager / lead | remove an employee | remove entries that I no longer need |
* * * |
project manager | remove a project | remove entries that I no longer need |
* * * |
manager / lead | search for employee details by name or ID | locate employee details without having to go through the entire list |
* * * |
project manager | search for project details by name or ID | locate project details without having to go through the entire list |
* * * |
project manager | assign employees to projects | update manpower allocation |
* * * |
project manager | un-assign employees to projects | update manpower allocation |
* * |
manager / lead | search for employees by skill sets | find employees with desired skills without having to go through the entire list |
* * |
project manager | search for projects by description | find projects that match a description without having to go through the entire list |
* * |
manager / lead | update employee details | update an entry without having to delete and re-create it |
* * |
project manager | update project details | update an entry without having to delete and re-create it |
* * |
user | hide private contact details | minimize chance of someone else seeing them by accident |
* * |
user managing many employees | categorize employees by their departments | organize employees based on their department |
* * |
user managing many employees | categorize employees into talent pools by skill sets | organize employees based on their skills |
* * |
user managing many projects | sort projects by their deadlines | focus on projects due earlier |
* * |
user that tracks project progress | list employees with project deliverables due soon | remind employees of their upcoming deliverables |
* * |
user that tracks project progress | list employees with project deliverables overdue | remind employees to complete overdue deliverables |
* * |
user doing manpower allocation | filter employees with certain skill sets | assign suitable people to projects |
* * |
user that recruits job candidates for projects | filter job candidates with certain skill sets | contact job candidates with skill sets sought after by the company |
* * |
frequent user | see a summary of upcoming interviews and project deadlines upon starting the App | stay updated without searching or filtering for details |
* * |
busy user | have most responses return within 200 ms | perform actions smoothly and quickly |
* |
user with many employees in the App | sort employees by name | locate an employee easily |
* |
user that has entered a lot of wrong data | reset the data to its default state | start over with fresh data |
* |
user with a lot of old data | archive outdated records | keep the database clutter-free and relevant |
* |
user that prefers short-form commands and keyboard shortcuts | use short-form commands and keyboard shortcuts to perform regular functions | perform actions more efficiently |
* |
user in-charge of employee up-skilling | track employees’ learning of new skills | monitor employees’ progress in learning new skills |
* |
user in-charge many manpower allocations | assign multiple employees to different projects using batch commands | manage manpower at scale |
* |
user returning after a long break | see recent changes made to the records | get back up to speed quickly |
* |
team lead | delegate manpower allocation tasks to other project managers | manage the team efficiently |
Use cases
(For all use cases below, the System is HRConnect
and the Actor is the user
, unless specified otherwise)
Use case: Remove an employee
MSS
- User requests to list employees.
- HRConnect shows a list of employees with their indices.
- User requests to delete a specific employee in the list by their index.
-
HRConnect deletes the employee.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. HRConnect shows an error message.
Use case resumes at step 3.
-
Use case: Remove a project
MSS
- User requests to list projects.
- HRConnect shows a list of projects with their indices.
- User requests to delete a specific project in the list by its index.
-
HRConnect deletes the project.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given index is invalid.
-
3a1. HRConnect shows an error message.
Use case resumes at step 3.
-
Use case: Assign an employee to a project
MSS
- User requests to list employees.
- HRConnect shows a list of employees with their ids.
- User requests to list projects.
- HRConnect shows a list of projects with their ids.
- User requests to assign a specific employee by their id to a specific project by its id.
-
HRConnect assigns the employee to the project.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
4a. The list is empty.
Use case ends.
-
5a. Either of the given ids is invalid.
-
5a1. HRConnect shows an error message.
Use case resumes at step 5.
-
Use case: Un-assign an employee from a project
MSS
- User requests to list projects.
- HRConnect shows a list of projects with their ids.
- User requests to see all members of a project by its project name.
- HRConnect shows a list of employees assigned to the project.
- User requests to un-assign a specific employee by their id from the selected project.
-
HRConnect un-assigns the employee from the project.
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
4a. The list is empty.
Use case ends.
-
5a. The given id is invalid.
-
5a1. HRConnect shows an error message.
Use case resumes at step 5.
-
Use case: Filter employees with desired skills
MSS
- User requests to list all employees with certain skills.
-
HRConnect lists all employees with all the skills selected.
Use case ends.
Extensions
-
1a. The list is empty.
Use case ends.
Non-Functional Requirements
-
Technical Requirement: The App should work on any mainstream OS as long as it has Java
17
or above installed. - Performance Requirement: Should be able to hold up to 1000 employees without a noticeable sluggishness in performance for typical usage.
- Performance Requirement: The App should respond to most commands within 200 milliseconds.
- Quality Requirement: A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
- Quality Requirement: A HR professional who has never used software to manage manpower should be able to learn basic operations like adding, deleting and assigning employees to projects within the first 10 minutes of reading the user guide.
- Fault Tolerance: The App should be able to handle corrupted or missing data without crashing.
- Documentation: The code should be well-documented so that maintainers new to the project can quickly understand and contribute to the codebase.
- Constraints: The App should be backward compatible with data produced by earlier versions of the App.
- Privacy Requirement: The App should comply with the Personal Data Protection Act (PDPA) in handling personal information.
- Notes about project scope: The App is not required to handle the actual firing / hiring of an employee or the completion or termination of a project.
Glossary
- Mainstream OS: Windows, Linux, Unix, MacOS.
- CLI: Command Line Interface - A way of interacting with a computer system by inputting lines of text. The keyboard is primarily used.
- GUI: Graphical User Interface - A way of interacting with a computer system where graphical elements such as windows, buttons, and menus are used.
- Private contact detail: A contact detail that is not meant to be shared with others.
- HR: Human Resources - A department responsible for finding, hiring, and training employees.
- Job candidate: An applicant who is being considered for a job. The applicant is not yet an employee.
Appendix: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
-
Initial launch
-
Download the .jar file and store it in an empty folder.
-
Open a command terminal, use the command
cd [folder path]
to navigate into the folder you put the.jar
file in, and use the commandjava -jar HRConnect.jar
to run the application.- Expected: Shows the GUI with a set of sample contacts, projects, and assignments. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the .jar file.
- Expected: The most recent window size and location is retained.
-
Deleting an employee
-
Deleting an employee while all employees are being shown
-
Prerequisites: List all employees using the
listemployees
command. Multiple employees in the list. -
Test case:
delete 1
- Expected: First contact is deleted from the list. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated.
-
Test case:
delete 0
- Expected: No employee is deleted. Error details shown in the status message. Status bar remains the same.
-
Other incorrect delete commands to try:
delete
,delete x
,...
(where x is larger than the list size)- Expected: Similar to previous.
-
Command history
-
Navigating through command history with the up/down arrow keys
-
Prerequisites: Enter several different commands (e.g.,
listemployees
,addproject pn/Project X pid/3
,delete 1
), so that there is a history of commands available. - Test case: Press the up arrow key (↑) in the command box.
- Expected: The previous command appears in the command box.
- Test case: Press the up arrow key (↑) repeatedly to cycle through older commands.
- Expected: Each press navigates one step back in the command history. The earliest command is reached when pressing the up arrow no longer changes the displayed command.
-
Test case: Press the down arrow key (↓) to navigate forward in the command history.
- Expected: Each press navigates one step forward in the command history. The most recent command is reached when pressing the down arrow change command box back to blank.
- Other incorrect delete commands to try:
delete
,delete x
,...
(where x is larger than the list size)
- Expected: Similar to previous.
-
-
Persisting command history across sessions
-
Prerequisites: Ensure there are commands in the history from a previous session.
-
Close the application and re-launch it by running the .jar file again.
-
Test case: Press the up arrow key (↑) in the command box.
- Expected: Commands from the previous session(s) appear, allowing navigation through the command history from earlier sessions.
-
-
Testing command history limits
- Enter 50 commands in succession (e.g.,
listemployees
,delete 1
, repeated or varied).- Expected: Only the 50 most recent commands are saved. Attempting to access a 51st older command with the up arrow key will not be possible.
- Enter 50 commands in succession (e.g.,
Other commands
-
Using / Testing other commands in HRConnect
- Refer to the User Guide “Features” section for an exhaustive list of commands.
- Valid command formats, command examples, parameter limitations, and expected outputs are provided.
- Enter the commands with the provided format and parameter limitations.
- Expected: The output performs the same as the listed expected output.
- Enter the commands not following the provided format or violating parameter limitations.
- An appropriate error message is thrown.
Saving data
-
Dealing with missing/corrupted data files
- With the app open or closed, find the data file in the folder you placed the .jar file, as
data/hrconnect.json
. - Delete the file.
- If the app is not open, launch the app by double-clicking the jar file.
- Create or delete any Employee, Project, or Assignment.
- Expected: A new data file is created at
data/hrconnect.json
containing the information in the app at the time.
- Expected: A new data file is created at
- With the app open or closed, find the data file in the folder you placed the .jar file, as
-
Transferring data
- Prerequisites: Make some changes to the sample data (so it is different from the default entries, e.g.
addproject
). - Copy the data folder at
data
(includinghrconnect.json
inside) into an empty folder. - Copy the .jar file into this same folder (not into
data
!). - Run the .jar file.
- Expected: The changes you made are still displayed in the app.
- Prerequisites: Make some changes to the sample data (so it is different from the default entries, e.g.
Appendix: Effort
HRConnect is based on the AddressBook Level-3 (AB3) (UG, DG).
Challenges and Difficulty
Both AB3 and HRConnect deal with their stored data as entities (Person
for AB3, Employee
, Project
and Assignment
for HRConnect).
However, HRConnect ended up as a significantly larger project due to the multiple entities involved (instead of only one). HRConnect also ended up more complicated in general due to the interactions between these entities. For instance, Assignment represents a link between an Employee and a Project, and we also had to deal with handling them in case of deletions to Employees or Projects.
Integration was also a challenge at the start, mainly due to some members’ unfamiliarity with GitHub and Git. There were a few merge conflicts that we were able to resolve. However, we were able to mitigate much of the pain points by splitting the work up into relatively defined portions.
Reuse
In the earlier stages of the project, some effort was saved by reusing certain parts of AB3 code. For instance, the code for adding and deleting projects was a heavily modified version of the original AB3 commands.
Due to the increasing size of our project, we have since refactored certain parts (e.g. all three list
commands) for better code structure.
Achievements
We had a clear and defined vision of the requirements and features from the start, which helped with executing the implementation (coding) portion of the project.
We are happy to say we were able to match all of our milestones, even the earlier ones.
We were also able to achieve a high level of test coverage for each Pull Request, and our features are confirmed to work well for the average target user so far.